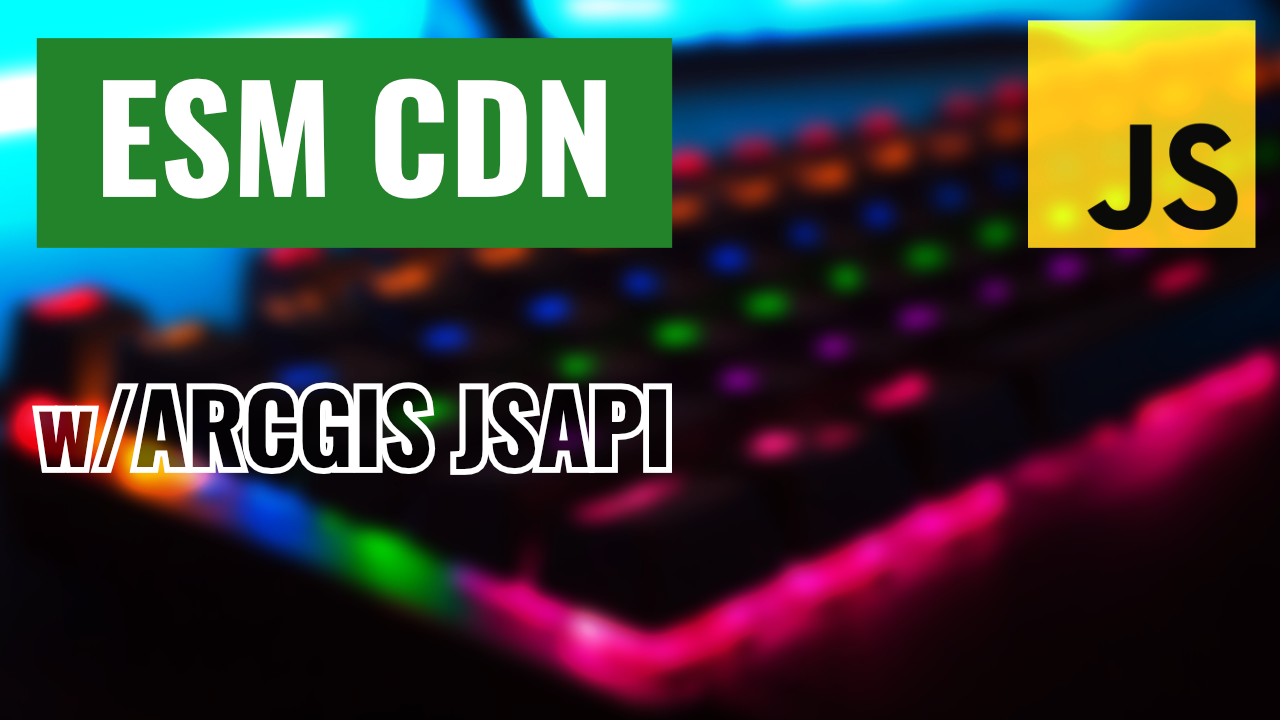
ArcGIS JSAPI ESM CDN
The latest version of the ArcGIS API for JavaScript introduced the release of the ES Modules for the API. I’ve been pretty excited about it, mainly because it makes the API more accessible to developers and makes it easier to use in different build environments.
Another option in using the API is via the ESM CDN.
Before I dive in, let’s be very clear. This should only be used for prototyping purposes. Test out some ideas, demo them, then take those ideas and build a production app in webpack or something. Please. Listen to me.
Ok, so ES Modules can be used across all the browsers that count. You can use them by add a script tag with a type of module.
<script type="module"></script>
Once you do that, you can now start loading ES Module JavaScript files into you app.
<script type="module">
import ArcGISMap from "https://js.arcgis.com/4.18/@arcgis/core/Map.js";
import MapView from "https://js.arcgis.com/4.18/@arcgis/core/views/MapView.js";
</script>
Notice that you import the .js
files using ESM natively. And you can load them directly from the CDN. After that, you can write your code as you normally would.
<script type="module">
import ArcGISMap from "https://js.arcgis.com/4.18/@arcgis/core/Map.js";
import MapView from "https://js.arcgis.com/4.18/@arcgis/core/views/MapView.js";
const map = new ArcGISMap({
basemap: "topo-vector"
});
const view = new MapView({
map,
container: "viewDiv",
zoom: 6,
center: [-118, 34]
});
</script>
This is a great way to test stuff out. Now, why would you not want to use this for production? Because this sample app loads over 400 JavaScript files. It’s unbuilt, unoptimized code. When built as a production application, it’s only a handful of files. But it’s a great way to get familiar with the API! You can check out a demo of this project here.
You can check out a video with more detail below!