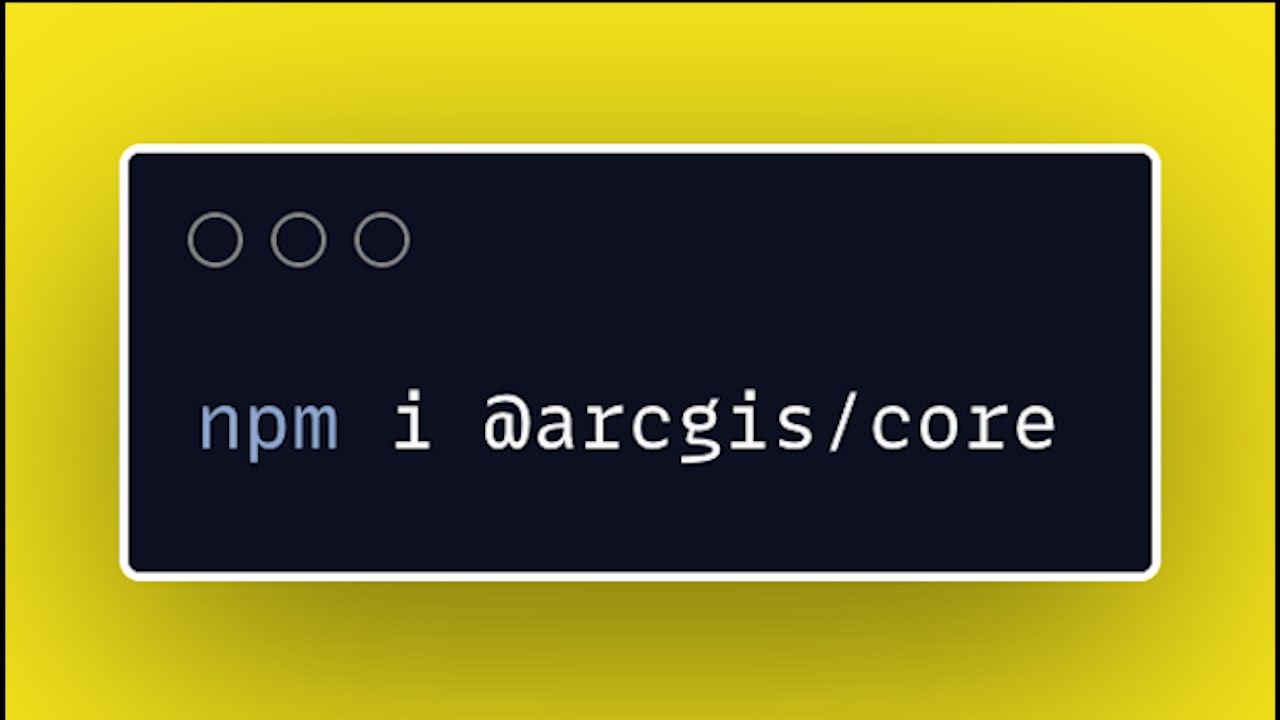
Using ES Modules with the ArcGIS JSAPI
What are ES Modules and Why should you care
EcmaScript Modules or ES Modules allow you to load JavaScript as needed and on-demand in your applications. Sound familiar? cough, cough. I’m just saying. ES Modules are a modern way of building web applications. They’re supported in most modern browsers and a variety of build tooling such as webpack and rollup.
What this means for the ArcGIS JSAPI
The ArcGIS API for JavaScript has a lot of features. Like a lot. It not only supports the ArcGIS Platform, but includes a multitude of visualization options, even 3D! All from a single library. This means, the whole of the library is pretty large. Historically, to make this fast for developers, the API has used AMD and AMD build tooling to optimize its use. Combine this optimized build with a cached CDN and it is already plenty fast and performant. The drawback is that it can become difficult to integrate the API with other frameworks and build tools. This is why the old version of the @arcgis/webpack-plugin
and esri-loader exist.
Now that the API is being released with and ES Modules options via @arcgis/core
, you don’t need those other options integrate the API with your React or Angular project anymore. You can npm install @arcgis/core
and start using it right away. This is huge for current and new ArcGIS web developers.
Show me the goods
There are a variety of samples using ES Modules you can explore. In your project, once the API is installed, you can begin adding some awesome Maps to your application.
import WebMap from '@arcgis/core/WebMap';
import MapView from '@arcgis/core/views/MapView';
const map = new WebMap({
portalItem: {
id: 'myfavoritemap'
}
});
const view = new MapView({
map,
container: 'viewDiv'
});
There’s no need for a plugin or special loader. It just works. There is one thing you need to do in your app. You need to copy the @arcgis/core/assets
folder to your build directory for your application. This assets
folder has all the fonts, images, styles, and worker files used by the API. This is where the latest release of the @arcgis/webpack-plugin
can be useful. It will handle copying the assets folder, and even filter out some files not needed at runtime, like sass
files and few more. You can even filter our language files you might not need in your application. However, I typically always recommend you just copy all the language files over. Even if your app doesn’t support multiple locales, the API does, so it can still display the proper language for your users.
Maybe you want to lazy-load some modules in your application. This is useful if you don’t need to display a map right away.
async function loadMap() {
const [
{ default: WebMap },
{ default: MapView }
] = Promise.all([
await import(`@arcgis/core/WebMap`);
await import(`@arcgis/core/views/MapView`);
]);
const map = new WebMap({
portalItem: {
id: 'myfavoritemap'
}
});
const view = new MapView({
map,
container: 'viewDiv'
});
}
This loadMap
function will dynamically load the modules needed to display the map. Talk about modern JavaScript!
You can watch me fumble through a demo below if you like!