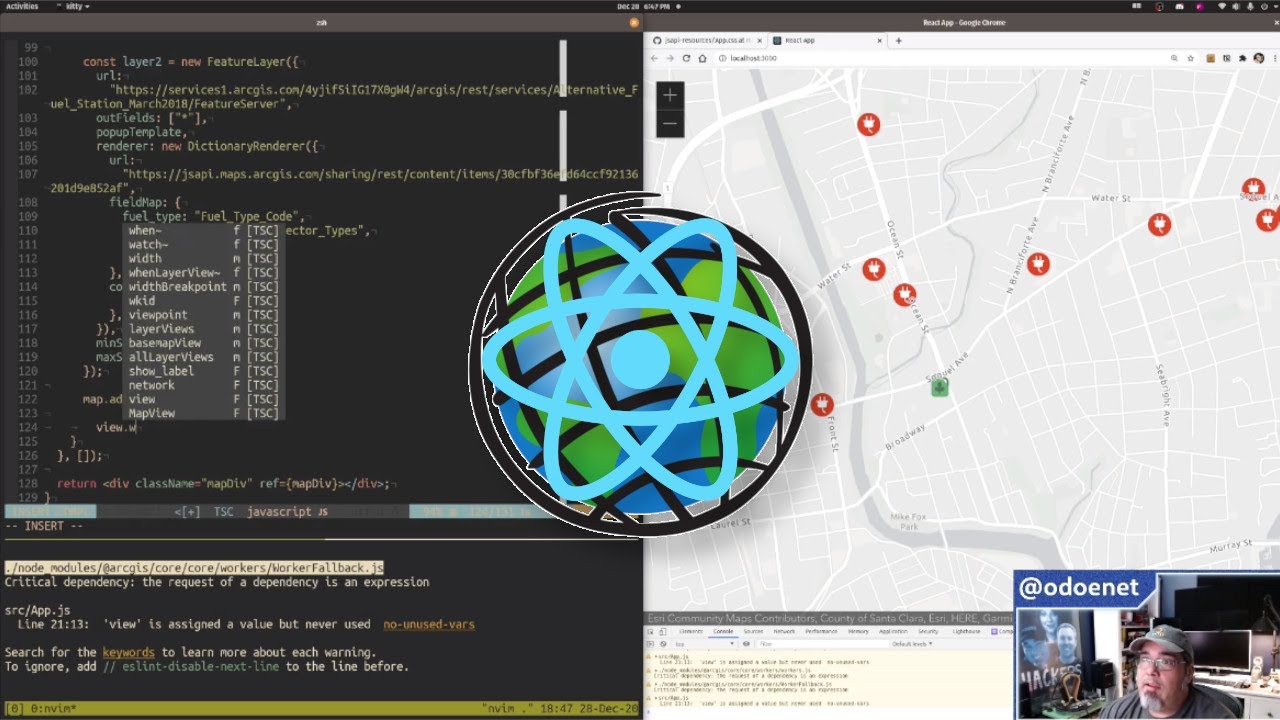
ArcGIS ESM with create-react-app
It’s never been easier
create-react-app is useful for developers to get started quickly building applications with React. The one drawback, or delight, depending how you look at it, is you don’t have access to the webpack config. That is unless you eject, which I’ve heard is something lots of users end up doing.
If you want to use @arcgis/core
with create-react-app, follow these steps.
npm install @arcgis/core
npm install --save-dev ncp
- Add a new npm script -
"copy": "ncp ./node_modules/@arcgis/core/assets ./public/assets"
npm run copy
- Update the browserlist in
package.json
// package.json
{
"browserslist": {
"production": [
"last 1 chrome version",
"last 1 firefox version",
"last 1 safari version"
],
"development": [
"last 1 chrome version",
"last 1 firefox version",
"last 1 safari version"
]
},
}
- Import the css in the
index.js
-import '@arcgis/core/assets/esri/themes/light/main.css';
That’s the core of it. I’ve mentioned this a few times, but the key thing to remember when using @arcgis/core
is you need to copy the assets
folder into your build directory. In the case of create-react-app, you copy them into the public
directory, and it will copy anything in public
to your deployed folder.
You should update the browserlist
because the ArcGIS JSAPI doesn’t support older browsers, and create-react-app tries to inject some babel helpers into code in the node_modules. I’m not sure why, but it breaks the build. You can do some babel stuff to get es5 support in there, but create-react-app doesn’t expose that configuration ¯\_(ツ)_/¯
So you can set the browserlist
and it won’t break.
Once that is done, you can start using the API like any other library.
import React, { useRef, useEffect } from "react";
import ArcGISMap from "@arcgis/core/Map";
import MapView from "@arcgis/core/views/MapView";
import "./App.css";
function App() {
const mapDiv = useRef(null);
useEffect(() => {
if (mapDiv.current) {
const map = new ArcGISMap({
basemap: "gray-vector",
});
const view = new MapView({
map,
container: mapDiv.current,
extent: {
spatialReference: {
wkid: 102100,
},
xmax: -13581772,
xmin: -13584170,
ymax: 4436367,
ymin: 4435053,
},
});
}
}, []);
return <div className="mapDiv" ref={mapDiv}></div>;
}
export default App;
There is a sample application you look at for more details in this Esri github repo.
Watch me try to remember how to React in this video!