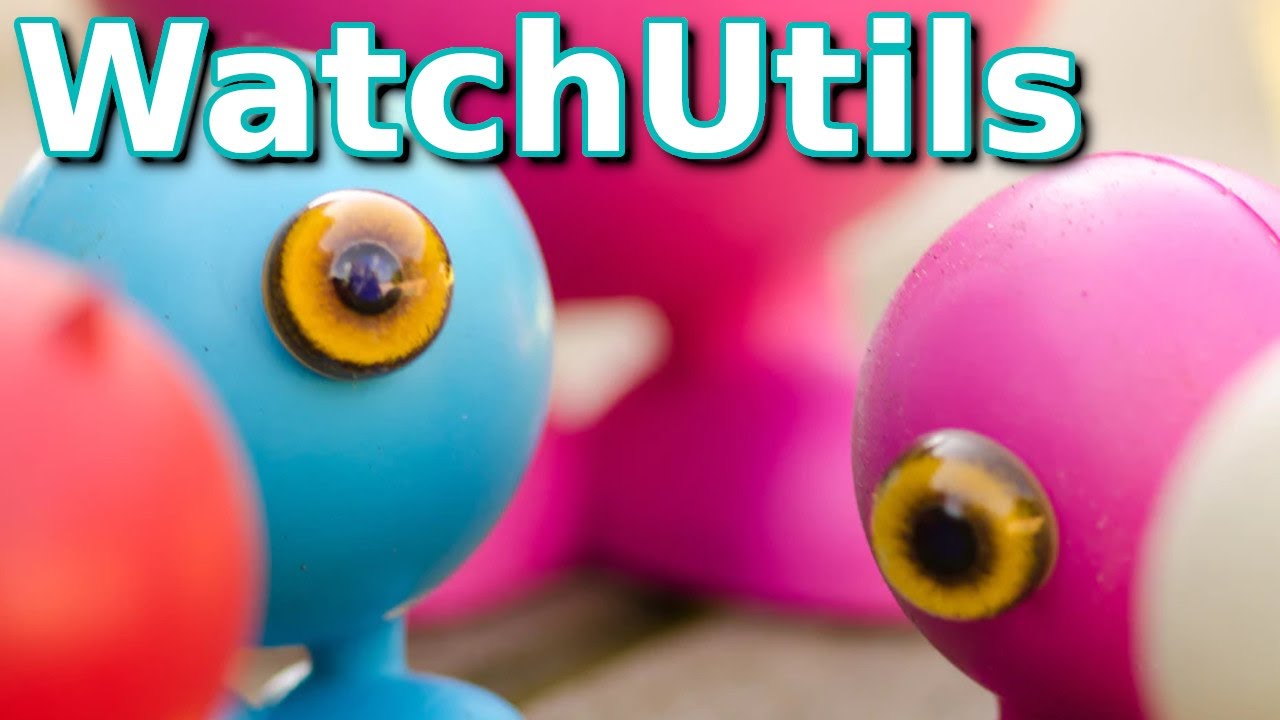
Who watches the WatchUtils
Watching the watchers
A key concept of working with the ArcGIS API for JavaScript is a basic understanding how properties work in the API, and how to watch for property changes. The API utilizes JavaScript Accessors, with a little sugar on top that allows for watching when those properties change.
Rather than rely on the API to provide detailed events for every little thing you’re interested in, you can just watch for specific properties to change. The most basic would look something like this.
view.watch('scale', (newScale, oldScale, propName, target) => {
if (newScale > oldScale) {
console.log('You got a big scale!');
}
else if (newScale < oldScale) {
console.log('You have a smaller scale!');
}
});
As you can imagine, there’s a lot you could do here. You can check if values are true or false, defined or not, maybe you only care about capturing this change one time. The watch methods also return a watchHandle with a remove method.
const handle = view.watch('ready', (ready) => {
if (ready) {
handle.remove();
}
});
There are some really useful patterns you can use here. Luckily, the API comes with a helper module specifically to work with properties using watchUtils.
watchUtils
I use watchUtils all the time. The ones I use the most are probably whenFalseOnce, whenTrueOnce, and once. The interesting thing about the WhenSomethingOnce methods is they are promise based. Since they only happen once, it makes sense.
A really interesting one is pausable. As the name says, you get a handle that you can pause and then resume.
const handle = pausable(view, 'scale', (scale) => {
handle.pause();
whenTrueOnce(view, 'stationary').then(() => {
console.log(view.scale);
handle.resume();
});
});
This little code snippet will capture a scale change, wait until the map view is stationary, meaning the navigation is done, then log the views current scale. This is the kind of problem you might encounter in your own applications, so it’s useful to know how to use watchUtils to handle it.
You can see more details of how this works in the video below.