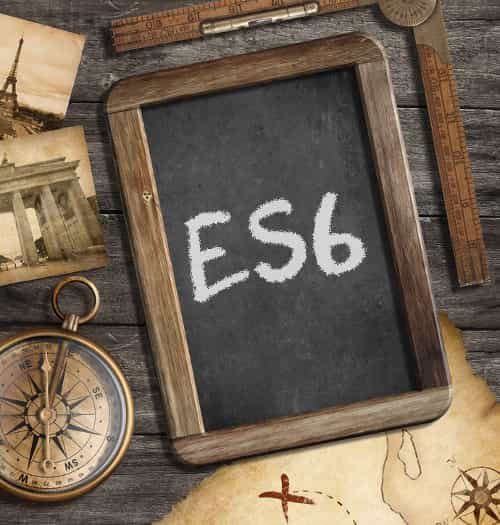
Use ES2015 with ArcGIS API for JavaScript
At the most recent Esri Developer Summit, I did a presentation on using ES6 for ArcGIS JavaScript development. ES6 is now referred to as ES2015, but I’m still not use to calling it that. I realized recently that I had not really discussed ES6 other than using it with a Leaflet project. So today, I thought I would walk through how to convert an existing application to ES6/ES2015 and how you can transpile your code on the fly to ES5 so that it runs in your browser.
Build tools
ES6 standards are not yet finalized, so browser support is still up in the air. Firefox supports some features and even Chrome has some limited support. This is why tools like Babel are so popular. Babel will convert your ES6 code to ES5 compatible JavaScript that will work in modern browsers today. This means your code base will be ready for when ES6 is universally supported. But let’s be honest, how much of the code that you write today do you expect to support until ES6 is in all browsers. What we really want is to get all the practice we can in writing ES6 so we are fully prepared for when it is ubiquitous across all browsers. It’s all about keeping up.
I’d suggest using gulp as a build tool for its simplicity in setting it up, but you could also use Grunt or any other build tool you’d like. We’ll set up these build tools later. I’m going to take an existing project and convert it to ES6, then when that’s done, use gulp with babel to convert it to ES5.
Import you heart out
One of the neat features of ES6 is the support for modular JavaScript files. You can now import files into other files, similar to CommonJS or AMD modules, but built into the browser. Let’s start with a simple one.
You should be able to follow along pretty easily here with what it is happening. Instead of using the define method to bring in external modules, you use the import keyword to designate the modules. When you use babel to transpile to ES5 there will be an option to turn this into an AMD module and it will use the deifne method in its output. That’s right, you can transpile your ES6 to commonjs require style or AMD define style. We’ll get to that later. Let’s look at another one.
There’s a couple of things happening here. First is that instead of returning the module, we are using the syntax export default, which means you are exporting the default method or object from this module. This is how you can make your module available to be _import_ed by other modules. You can read more about this module behavior here. Also notice how for postCreate(), we didn’t have to use the function keyword. This is a little syntactic sugar in ES6 that works for functions that are part of classes or objects.
Speaking of classes…
In this case, we are now using an ES6 class. Don’t worry, for all intents and purposes, JavaScript is still a prototype language, but classes were introduced in ES6 to simplify some behavior… I think. So instead of using dojo/_base/declare to define our module, we just use a class that extends Stateful and we can use the constructor method to set up our default properties. Notice the use of super() here. Since we are this in the constructor to set the defaults, we need to call the constructor of the class we are extending, you do this via the super method. You can read more about classes here.
I won’t go over all the module changes here, but you can check out the full project on github.
Take a gulp
Let’s set up a simple gulpfile to handle the transpiling of your JavaScript code for you.
What this gulpfile is doing is using the JavaScript files in the src directory, running them through babel with the parameters designating you want amd output. That’s right, you can write your code in ES6/ES2015 and output to either AMD or CommonJS, whichever works for you, but in our case using Dojo, we want AMD. I also added a task to copy widget template files to the dist directory. This is a basic setup, but it’s a good start to writing your code in modern ES2015 today. There is even a watch task that will monitor your code and do the transpile as you save changes to your code.
Get coding
There’s a whole lot more to ES6/ES2105 than what I covered here. You can find more resources in the links in my presentation slides. Babel provides some great info as well.
Don’t be afraid to start using ES6/ES2015 for your ArcGIS JavaScript development today. There’s no point in really putting it off as it will be available soon enough (at least by end of 2015 if things stay on track). Go forth and code.