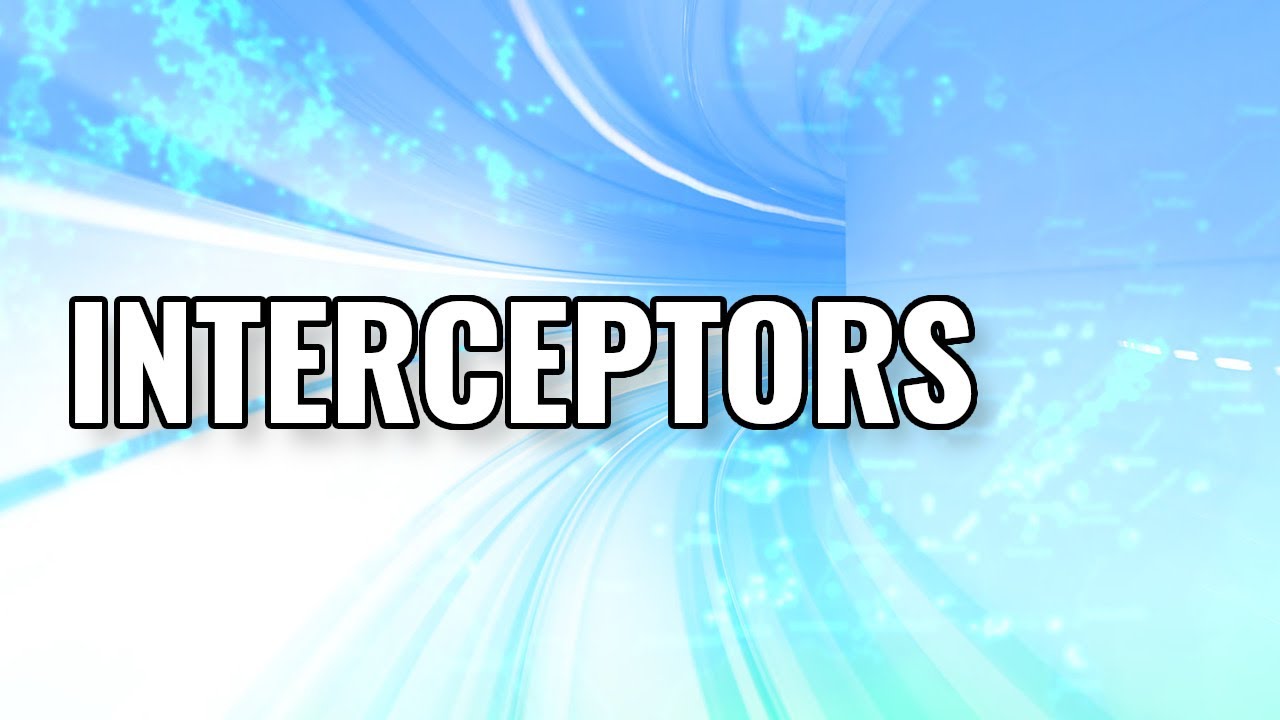
Filter GeoJSON in your ArcGIS Apps
Filter GeoJSON in your ArcGIS Apps
GeoJSON is a popular data transport format for spatial data. You can think of it as the shapefile of the web. It’s not a storage format, but I won’t get into that. A lot of agencies and organizations will provide a GeoJSON file that users can download and use in their own applications. This is super helpful and a really simple way to share data. Downside is, most of the time, this is a static file that might get updated on a regular basis. Yes, a web API can be written that can return results as GeoJSON, but most are just simple files. This means you can’t really filter the data out before you use it.
The ArcGIS API for JavaScript can load GeoJSON files pretty easily using a GeoJSONLayer. Once it’s a GeoJSONLayer, you can edit it, query features, even run statistics and use advanced visualizations and filtering with FeatureEffects.
You might want to do something to the GeoJSON before you actually consume it in your application. Maybe grab only the first 500 features or do some crazy stuff to add and populate new fields. This is the kind of task you can accomplish using a request interceptor.
Let’s assume you want to load some earthquake GeoJSON data.
const layer = new GeoJSONLayer({
url: `https://earthquake.usgs.gov/earthquakes/feed/v1.0/summary/all_month.geojson`,
id: "buildingPoints",
title: "Indoor Maps Locations",
displayField: "siteName",
renderer: {
type: "simple",
symbol: {
type: "simple-marker",
style: "square",
color: "blue",
size: "8px"
}
},
geometryType: "point"
});
Maybe you only care about grabbing the first 500 earthquakes. You can accomplish this using an after()
method in your request interceptor.
esriConfig.request.interceptors.push({
urls: 'https://earthquake.usgs.gov',
after(response) {
const geojson = response.data;
geojson.features = geojson.features.splice(1, 500);
response.data = geojson;
return response;
}
});
Note, if you are using an earlier version of the ArcGIS API for JavaScript, you might need to parse the response data using TextDecoder and TextEncoder. You can find more details in this video.
Remember, if there is something you want to do in the ArcGIS API for JavaScript that may not seem straightforward, there is probably a way to accomplish it. Even using static GeoJSON files.
You can find a demo of this application below.